https://github.com/sevkeifert/text-utils/blob/master/svi
A while back I needed a really simple way to encrypt a password file on the command line. I wanted to be able to write quick, simple scripts, with no more than one line of code handling the file encryption part. So, in Linux or Windows (using cygwin), I used these aliases:
# for simple encryption methods, use: # echo "text" | encrypt # echo "U8askj123lkjasdflkjasdlkjargoi+/==" | decrypt alias encrypt="openssl aes-256-cbc -a -salt" alias decrypt="openssl aes-256-cbc -d -a -salt"
Then other quick shell scripts can easily encrypt/decrypt files as needed.
However, while this works, it was still a nuisance to actually edit and maintain the encrypted files. Here's an alternate approach that will work under Linux or Windows (using cygwin). It wraps the classic vi text editor, or any editor for that matter, in a shell script that opens and saves files in any standard encryption format (like aes 256), which can then be decrypted by other script utilities. Also, it will save an encrypted snapshot of the file every day it's updated in case you mangle the password or file.
Here's the bash script, which can be saved to /usr/local/bin/svi
#!/bin/bash # A simple secure vi editor, for aes encrypted files # file name passed in as argument, for example # svi FILENAME # to open file as read only # ln -s svi sview # sview FILENAME # GPL # type of encryption cipher=aes-256-cbc # where temp files will be decrypted tmp=/tmp/ # create tmp dir/file me=`basename $0` dir=`dirname "$tmp$1.tmp"` mkdir -p "$dir" touch "$tmp$1.tmp" chmod 600 "$tmp$1.tmp" # decrypt to tmp if [[ -e "$1" ]] then if openssl $cipher -d -a -salt -in "$1" > "$tmp$1.tmp" then if [[ ! -s "$tmp$1.tmp" ]] then echo "WARNING: decrypted an empty file, exiting." exit fi else echo "WARNING: bad password, exiting." exit fi fi if [[ $me == 'sview' ]] then # open file read only vi -n -R "$tmp$1.tmp" else # open file for editing vi -n "$tmp$1.tmp" # re-encrypt after exiting vi if [[ -s "$tmp$1.tmp" ]] then touch "$tmp$1.aes" chmod 600 "$tmp$1.aes" while [[ ! -s "$tmp$1.aes" ]] do openssl $cipher -a -salt -in "$tmp$1.tmp" > "$tmp$1.aes" done # make backup, replace old if [[ -s "$tmp$1.aes" ]] then if [[ -s "$1" ]] then cp -pf "$1" $1.$(date "+%Y-%m-%d").bac fi mv -f "$tmp$1.aes" "$1" fi fi fi # cleanup shred -z -u "$tmp$1.tmp" rm -f "$tmp$1.aes"
Then for example, to open or create a new encrypted text file, use:
svi YOUR_FILE
Disclaimer
Overall, this is a reasonably simple and secure method for protecting files, in the case a laptop is lost or stolen. The strength is that these files can be transferred across or stored on insecure media.
As for weaknesses though, this does create a decrypted copy of the file on the local computer while it is being edited. And the temp file, even if deleted (unlinked), may leave an image on the local disk. Another weak point is that insecure memory could be flushed to swap space on the hard disk.
And, as with any encryption cipher, it can be always broken with the old "wrench" method.... :)
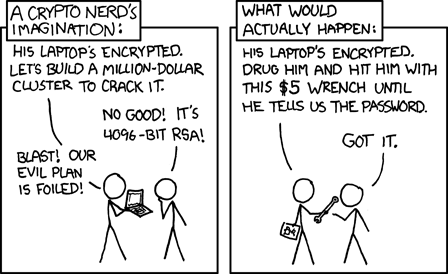
(comic courtesy of xkcd)
1 comment:
i am always looking for some free stuffs over the internet. there are also some companies which gives free samples.
encryption
Post a Comment